Rationale
As part of my master’s thesis, I’m comparing and evaluating several cross-platform mobile frameworks. I also wanted to have PhoneGap (now called Cordova) in the comparison, but since it does not include a UI library, I decided to combine it with Sencha Touch 2 for that purpose. Why not jQuery Mobile you may ask? Rhodes includes jQuery Mobile, and I covered Rhodes already in my comparison – and I don’t want to compare too similar frameworks. Also, Sencha Touch only includes dependencies that are actually used (concatenated into a single file), thus it seemed worthwhile to check its performance. And yes, Sencha Touch comes with native packaging for Android, but 1) that didn’t work for me, 2) does not include the device functionality offered by PhoneGap and 3) should be as performant as the PhoneGap app wrapper since it uses the platform’s web view component.
Update: Sencha Touch 2.1 and Sencha Command Utility
Somebody commented that people are having trouble when using the newer Sencha Touch 2.1 SDK. This article walks you through setting up version 2.0. Since I don’t use Sencha Touch for myself and the Sencha team might change their mind again to reinvent their own build toolchain, I will not update the whole article. However, I found that there are only few differences introduced by the new “Sencha Command utility” (replacing “SDK tools”) and build system, and my example application below works fine with Sencha Touch 2.1 if you consider the following differences:
-
sencha app create
is nowsencha generate app
-
sencha app build testing
will not consider the argument-d android/assets/www
anymore but looks into thebuild.xml
file to find the build output directory. It defaults to<project dir>/build/<project name>/testing
. -
Unchanged: The
sencha app build
process still happily returns 0 in case of errors, so the wrapper script is still necessary. -
You will have to adapt the wrapper script or your
build.xml
file to output the application into theandroid/assets/www folder
.
Let’s get going
First of all, I’m using Eclipse to package the Android app, so make sure you have everything
installed to create a simple Android project. In this article, I’m
using Windows but it should work the same way on Linux and others. You will also need the
Sencha Touch SDK and tools –
ensure that the sencha
command works and that it is
always on the PATH (on Windows, just restart your computer). At the time of writing, the Sencha Touch SDK version was
2.0.1.1 and the Sencha Touch SDK tools version was 2.0.0-beta3. For some black magic build automation, you will also
need Python (UPDATE: I use 2.7, but it should also work with 3.x).
The directory structure
Since the Android project will later go in a subdirectory, let me first explain the directory structure that our application will have:
-
AndroidSencha
-
android
-
assets
-
www
-
-
libs
-
res
-
src
-
.project (and other Eclipse Android project files)
-
-
app
-
resources
-
sdk
-
.senchasdk
-
app.js
-
app.json
-
cordova-x.y.z.js
-
index.html
-
sencha_wrapper.py
-
The AndroidSencha
directory contains the app scaffolding created by Sencha Touch, i.e. app
(models, stores, views,
controllers), resources
(CSS, images), sdk
(necessary Sencha Touch SDK files), .senchasdk
(points to the SDK),
app.js
, app.json
, cordova-x.y.z.js
and index.html
. The android
folder will be created manually and contains
our Eclipse project. And sencha_wrapper.py
is my wrapper script for the sencha
command that will be explained later.
If this does not make sense to you, check out the finished application at Github or just bear with me in the rest of the article.
Create the Sencha app
cd "/path/to/downloaded/sencha/sdk"
sencha app create AndroidSencha "/path/where/you/want/the/app/AndroidSencha"
The command should now copy/create some files and directories.
Create the Android project in a subdirectory
Now it’s time to do some bootstrapping for the Android part. In the newly created application
directory (the one that contains app.js
), create a folder named android
and create a new
Android project there using Eclipse:
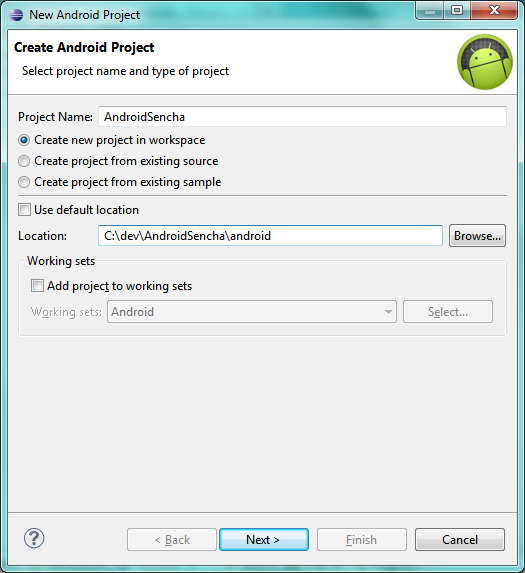
Note: I use Android 2.3.3 (SDK version 10) as build target and the package name org.dyndns.andidogs.androidsencha
.
You might run into the error "Can’t find variable: Ext" or similar if you use the 2.2 or older emulator (see
here).
Set up PhoneGap
PhoneGap is set up almost as usual with Android projects, following the
official guide,
but some steps differ a bit. In the android
folder:
-
Create the folders
/libs
and/assets/www
-
Copy
cordova-x.y.z.jar
to/libs
and add it to the build path using Eclipse -
Copy the
xml
folder from PhoneGap to/res
-
Make the changes to the main activity (in my case
AndroidSenchaActivity
) -
Different and optional: Add
setIntegerProperty("loadUrlTimeoutValue", 60000);
before thesuper.loadUrl
call in case you run into timeout problems! -
Make the changes to
AndroidManifest.xml
-
Different: It is not necessary to put
cordova-x.y.z.js
and the sampleindex.html
file into/assets/www
, but you might want to do that and run the app on the emulator to see if the PhoneGap "Hello World" works!! Note that theindex.html
will later be overwritten automatically, so don’t change it in the/assets/www
directory, in fact don’t change anything there! (you will see later why)
Test if Sencha Touch is working
We have a main app directory for the Sencha Touch application and a subdirectory for Android. You should now check if the Sencha Touch application actually works. By default, it should contain a tab bar with two different views. Fire up a web server in that main directory and open it up in a browser (should be a Webkit browser, not Firefox). With Python, it’s as simple as:
python -m SimpleHTTPServer 8000
# Multithreaded alternative if you have Twisted installed:
twistd.py web --path . --port 8000
Note that it might take some time to load (especially with the single threaded SimpleHTTPServer of Python). It should look something like this:
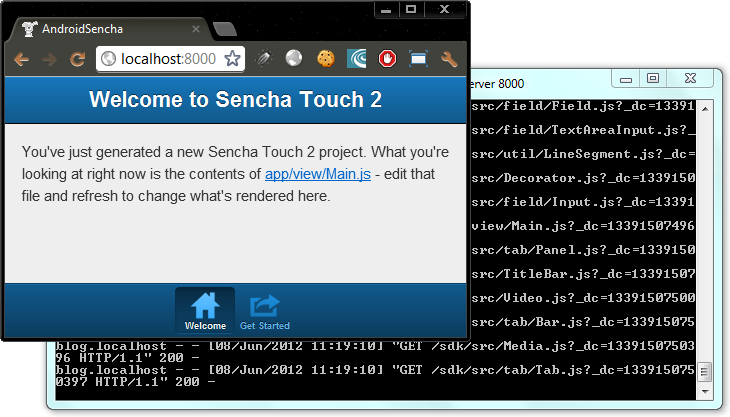
Important: The fact that it works in the browser does not mean it works on a mobile device/emulator. It took me a
while to find out that you have to change "logger": "no"
in app.json
to "logger": "false"
. Else you will get an
error like "Error: [Ext.Loader] Failed loading 'file:///android_asset/www/sdk/src/log/Logger.js', please verify that
the file exists at file:///android_asset/www/sdk/sencha-touch.js:7908".
PhoneGap working? Sencha Touch working? Time to combine them!
Including PhoneGap
First of all, add the PhoneGap script as dependency, so copy cordova-x.y.z.js
to the main folder and change app.json
to include it – therefore you only have to add it to the key "js".
"js": [
{
"path": "cordova-1.8.0rc1.js"
},
Building
Sencha Touch comes with the sencha
command line utility that can build an application, i.e. scan its dependencies, concatenate necessary files into a final app.js
, copy resources etc. What we want to accomplish is to put that build output into the Android app’s /assets/www
folder. And that’s why I said, don’t edit any files there because they will get overwritten.
I am using a simple builder configuration in Eclipse to run this command (will be explained below). Unfortunately,
it seems that Eclipse does not stop the build if the sencha
command returns an error – the sencha
command actually
always returns 0, but even if I wrap it with a script, Eclipse does not stop the build on non-zero return codes. Also,
when set up as builder, Eclipse hides the sencha
command output after completion (don’t know why?). That is a problem,
because if you have a syntax error or other mistake in your Sencha Touch app, then you will see only the loading
indicator and some unhelpful "[Ext.Loader] Failed loading…" error in LogCat once you try and start the app. I work
around this problem with a dirty hack wrapper for the sencha
command. In the main folder (the one with app.js
),
add the file sencha_wrapper.py
with the following content:
import os
import subprocess
import sys
def contains_errors(s):
return '[ERROR]' in s
def get_errors(s):
ret = ''
for line in s.splitlines():
if contains_errors(line):
ret += line + '\n'
return ret
print('Running Sencha command...')
try:
proc = subprocess.Popen(['sencha.bat' if os.name == 'nt' else 'sencha'] + list(sys.argv[1:]),
stdout=subprocess.PIPE,
stderr=subprocess.PIPE)
stdout, stderr = proc.communicate()
# Try to decode output to Unicode
stdout = stdout.decode('utf-8', 'replace')
stderr = stderr.decode('utf-8', 'replace')
if proc.returncode != 0 or contains_errors(stdout) or contains_errors(stderr):
return_code = proc.returncode or 1
sys.stderr.write('Command failed\n')
else:
return_code = 0
sys.stdout.write(stdout)
sys.stderr.write(stderr)
except Exception as e:
stdout = ''
stderr = ('[ERROR] Failed to execute sencha command, did you reboot and ensure the sencha command is always on the '
'PATH? (%s)' % str(e))
return_code = 2
# Eclipse does not seem to stop the build even for return codes != 1, so let's be a bit more cruel
with open(os.path.join('android', 'AndroidManifest.xml'), 'r+t') as f:
MAGIC = ('SENCHA BUILD FAILED, PLEASE CHECK FOR ERRORS AND RE-RUN BUILD (THIS LINE IS REMOVED AUTOMATICALLY IF '
'SENCHA BUILD SUCCEEDS)')
content = f.read()
magicPosition = content.find(MAGIC)
if magicPosition != -1:
content = content[:magicPosition].strip()
if return_code != 0:
content += '\n' + MAGIC + '\n' + get_errors(stdout + '\n' + stderr)
f.seek(0)
f.write(content)
f.truncate()
exit(return_code)
This script runs the sencha command with the passed arguments and checks if the string "[ERROR]" occurs in the output
(by the way, they also don’t use stderr as they should) and if so, writes these errors to the end of
AndroidManifest.xml
and thus stops the Android build process because that XML file is no longer valid. As I said,
a dirty hack. These lines are automatically removed once you correct the Sencha Touch app mistakes and run the script
again.
So let’s try that out. From the main folder, run python sencha_wrapper.py app build testing -d android/assets/www
.
If successful, it will show no errors and end with "Embedded microloader into index.html". Now go to Eclipse, refresh
the project (select project name and hit F5) and then run it. The app should work without problems (only the emulator
is slow as hell):
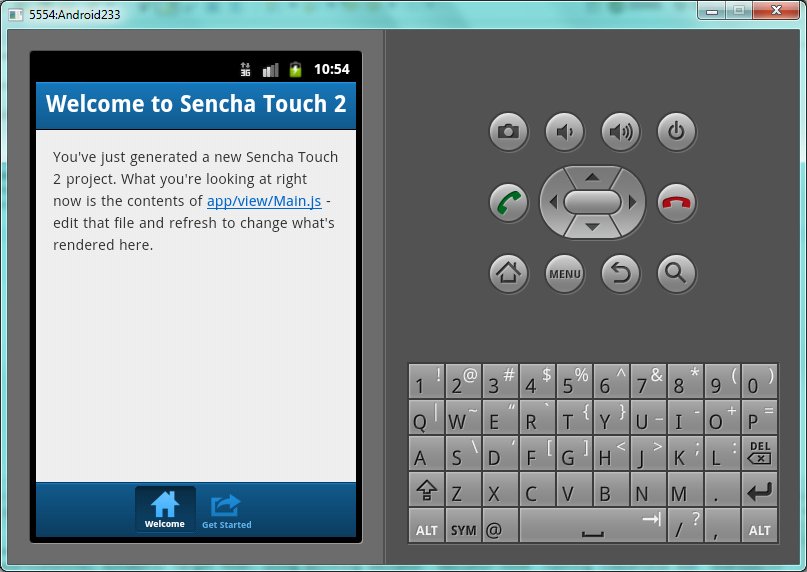
Build automation
Great progress! But of course you don’t want to use the command line and refresh manually every time you want to run your app, so let’s automate this. In Eclipse, right click the project, select "Properties" and then "Builders", "New…" and "Program". Configure it as follows (your Python path will vary):
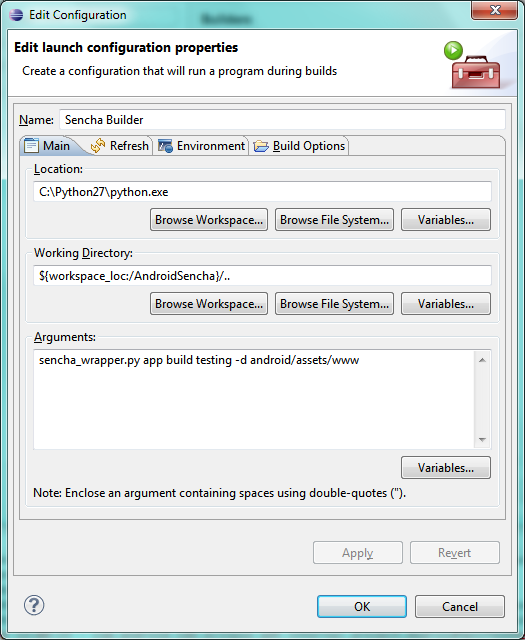
Click "OK" and use the "Up" button to move that builder to the beginning of the list:
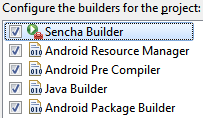
Ensure that AndroidManifest.xml
is refreshed after the script is run:
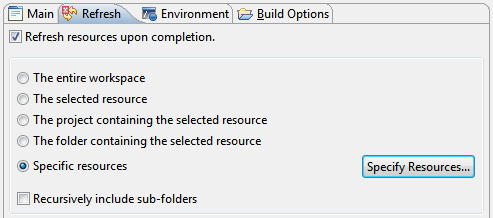
As a bonus, you can set up another builder called "Force recompile" that ensures that every time you click the run
button in Eclipse, the Sencha Touch app is recompiled and Eclipse rebuilds the Android app instead of bringing the
current intent to the foreground as it would normally do if it doesn’t recognize any changes (which it can’t because
Sencha Touch app changes are in the parent directory!). The builder is configured as follows, note that you will need
touch.exe
(equivalent for the touch command of Linux, for Windows you can use the one from
msysgit or the
gnuwin32 coreutils package):
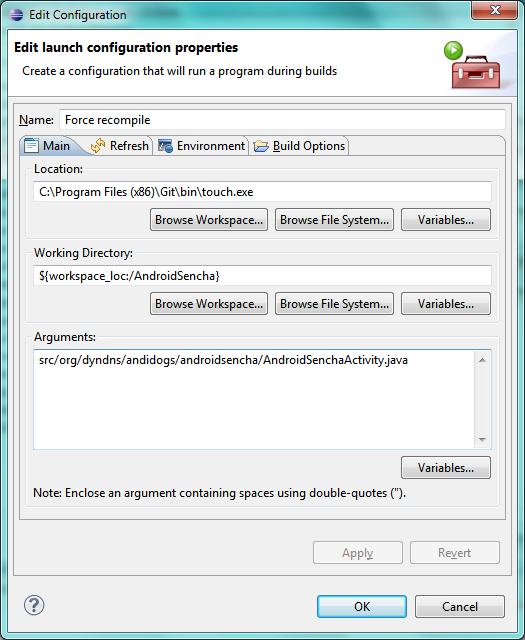
Make sure it’s located before "Android Package Builder":
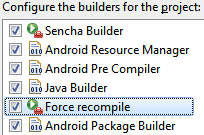
That’s it, now when you click the run button in Eclipse, the app should always be recompiled from the current Sencha Touch source code in the main folder.
Short test
Open app/view/Main.js
and replace the Getting Started content as follows:
html: "<a href=\"javascript:navigator.notification.alert('Congratulations, you are ready to work with Sencha Touch 2 and PhoneGap!')\">Click me</a>",
Run the application and when you click the link, you should get a native alert box:
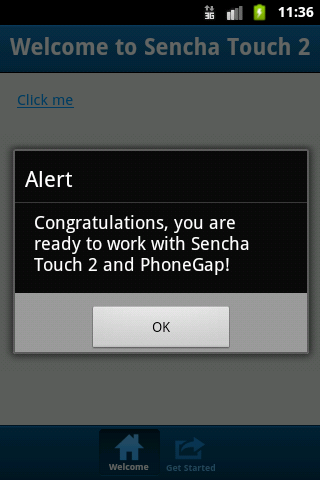
Finished!
So there you have it, two possibly great frameworks combined! Whether they really are that great – I will find out in my thesis while implementing a sample application using this combination. Then I shall see how it performs on my Huawei Ideos X3 (probably the slowest Android phone available). You can get the finished application at Github.
Some hints: Again, do not change anything in /android/assets/www
, but rather edit the code in the main folder
(app.js
and anything in app and resources). Mind that we used the command app build testing
in our builder – this
is cool for debugging because it leaves the JavaScript unminified. If you want to release your app, you should replace
testing
by production
.
Note also that PhoneGap might not be available as soon as Sencha Touch loads. I tried it in a painted
event and it
was not loaded yet. Since the sencha app build
command loads the application to find dependencies, you have to take
care that it can be loaded – using PhoneGap in startup code will produce errors, for example.
And watch out for stupid syntax errors:
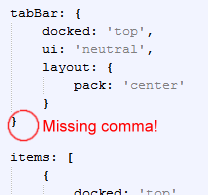
Feedback and further considerations
I’m happy to hear any feedback to this article. Just leave a comment or send me a mail. If someone finds out how to create a splash screen that hides when Sencha Touch is loaded, or how to use PhoneGap in startup code, let me know! Cheers!
Archived comments from old blog:
I saved those from the old system since this article is still popular, so the comments may be important. Commenting is no longer possible.
Two things though.
1. Maybe you should explain, where you get the touch.exe
2. Could you provide us a screenshot how your Eclipse project explorer looks like? I was a bit confused with all the folders :)
Also do you develop your SenchaTouch2 app in Eclipse?
Once again, Thanks a lot!
Cliff
one point-it may be useful to point out how you launch the apk in the emu as some phonegap examples use the RunAs > Android Appl(right click on project) and not the usual run configuration setup.
Other than that a great headsup for my compilation.Many thanks
I'm not really sure what you are saying here with Eclipse on OSX – can you explain that? The Android project (.project file) goes into the android subdirectory that you create manually.
I'am getting the following error.
CordovaLog(828): Uncaught TypeError: Cannot call method 'alert' of undefined
CordovaLog(828): file:///android_asset/www/index.html: Line 1 : Uncaught TypeError: Cannot call method 'alert' of undefined
Web Console(828): Uncaught TypeError: Cannot call method 'alert' of undefined at file:///android_asset/www/index.html:1
Do you help me? Thanks.
If i use one cordova,exec command, there fires a error that i use it before device ready.
I know that sencha touch fires after device ready, so i don't know wheres the Problem, did you know that Problem?
The funny thing is, that all works fine on iOS
If you need Cordova when the app starts, you may want to try a workaround. For example I'm hiding Cordova's splash screen when deviceready has fired:
// In global context
document.addEventListener("deviceready", function() {
console.log("phoneGapReady()")
phoneGapReady = true
console.log("phoneGapReady()~")
}, false)
// In app.js
launch: function() {
console.log("launch()")
function hideSplashScreen()
{
if(phoneGapReady)
navigator.splashscreen.hide()
else
setTimeout(hideSplashScreen, 100)
}
setTimeout(hideSplashScreen, 100)
// other stuff
console.log("launch()~")
},
"js": [
{
"path": "cordova-1.8.1.js",
"path": "sdk/sencha-touch.js"
},
written as follows
"js": [now it works.
{
"path": "sdk/sencha-touch.js"
},
{
"path": "cordova-1.8.1.js"
},
very thanks.
Please help.
The above error is occurring where as sencha-touch.js file exists, but the log\logger.js doesn't exist. FYI.. if that helps in answering my issue.
"logger"
value in the file app.json to "false"
as denoted in my article. If that doesn't work, please try false
without quotes. I think I read about a recent change in Sencha Touch and you have to use a normal boolean value instead of a string. Please let me know if that worked!
{"id":"2f1abb90-c085-11e1-8064-f3c7ed002926","js":[{"path":"sdk/sencha-touch.js","type":"js"},{"path":"app.js","bundle":true,"update":"delta","type":"js"}],"css":[{"path":"resources/css/app.css","update":"delta","type":"css"}]}
I don't see logger entry, am I missing anything?
I have another key
"buildOptions"
in that file, maybe you can try adding this:"buildOptions": {
"product": "touch",
"minVersion": 3,
"debug": false,
"logger": "false"
},
and then try which value works for
"logger"
.Which version of the Sencha Touch SDK are you using?
2. I tried adding the "buildOptions" tag and tried with all options "false and no" with and with out quotes. Here is the updated app.json file
{"id":"2f1abb90-c085-11e1-8064-f3c7ed002926","js":[{"path":"sdk/sencha-touch.js","type":"js"},{"path":"app.js","bundle":true,"update":"delta","type":"js"}],"css":[{"path":"resources/css/app.css","update":"delta","type":"css"}],"buildOptions": [{"product": "touch","minVersion": 3,"debug": false,"logger": "no"}]}
3. Sencha Touch version 2.0.1.1
Please let me know if there is anything else I should try.
Regards
Srini
"logger"
value.
06-29 18:36:18.754: E/Web Console(560): Uncaught Error: [Ext.Loader] Failed loading 'file:///android_asset/www/sdk/src/log/Logger.js', please verify that the file exists at file:///android_asset/www/sdk/sencha-touch.js:7908
Let me know if you think of anything.
Regards
Srini
"logger": "no"
just like I said, which you have to replace by "logger": "false"
. I don't know which JSON file you quoted above.And don't forget to add the builder configurations to the Eclipse project.
I am not following this line "And don't forget to add the builder configurations to the Eclipse project"
"logger": "false"
in the files of your ZIP file.With the "builder configurations", I mean the ones I mention in my article. Please read the article carefully. I didn't see in your Eclipse project that you set up these builder configurations. The first one is very important because it compiles the application and puts it into the Android project's assets/www folder. It is possible that you forgot that, and therefore your changes in <root folder>/app.json did not get copied to <root folder>/android/assets/www/app.json.
Thanks for your help and support. I will continue with the next steps in the article and will let you know how it goes.
I'm encountering a problem when following your guide.
When i have to combine phonegap and sencha i get an error from that it can not find the file index.html. Another issue is when launching the web server with python, i solved that by first starting in the AndroidSencha catalog and from there launch the python simpleserver. But when i have to combine phonegap and sencha i'm also starting in androidsencha catalog but it cannot find all the files. How is your python installation on windows? Mine is under C:\python27\ and AndroidSencha is C:\AndroidSencha\. The issue maybe already starts that i can't just type python in cmd, i have to be located inside the AndroidSencha catalog and run the python web server.
Any help would be appreciated. Thank you for a great tutorial by the way!
Let me know if that solved your issues.
launch
function in app.js – it's all in their tutorial. Just make sure you don't change index.html as they do, follow from the heading I mentioned above.
The problem was not testing the web server it is when i merge phonegap and sencha: python sencha_wrapper.py app build testing -d android/assets/www. I get an error: Failed loading your applicaition from: 'file:///C:AndroidSencha/index.html'. Try setting the absolute URL to your application for the 'url' item inside 'app.json'..I've tried setting the url but then it just show the index.html content. I'm running win7 x64 if it has any meaning.
I have ziped my project and placed at the following link https://docs.google.com/open?id=0B1h-jczMK2IBZXl1Tlo1Mkl4bXM
Only thing is I just commented out "Ext.Viewport.add(Ext.create('AndroidSencha.view.Main'));" so that other page will be loaded initially.
I have included logCat messages in LogCat.txt file, kindly let me know if I am doing anything wrong here.
Regards
Srinivas
Thanks for the help.
Regards
Srinivas
Please help in proceeding further.
Thanks And Regards
Srini
Regards
Srini
Is there such tutorial to package Sencha Touch 2 to Windows Phone?
If you have any references please help me.
Thanks and Regards
Srini
For Windows Phone, the process is quite similar. Don't know any tutorial, but it should work the very same way as I explained in this article, except that you would have to create a custom builder in Visual Studio that runs python sencha_wrapper.py app build testing -d
I just want to give this FYI.
Regards
Srinivas
I've still not been able to compile the project, (python sencha_wrapper.py app build testing -d android/assets/www). Just keeps saying that it can't find the index.html file. I've also looked around at other forums with similar problem, one suggested to not have the Sencha tools folder under Program Files due to the space in the folder name, but this didn't either solve my problem. I've also tried to set the absolut url path in the app.json file and still unsuccessful. Don't know what I've missed because it works on a mac but now on my Win7 x64. Any other suggestions would be apperciated. /Victor
spawn
calls in the sencha tool (sencha.js and probably other files in the SDK tools folder) and check whether the executed file actually exists. For example, bin\jsdb.exe is called from sencha.js.
I was also getting the same "Uncaught TypeError: Cannot call method 'alert' of undefined". I added "Cordova-x.y.z" in the head of index.html and that solved the problem.
Thank you :)
[1m[31m[ERROR][39m[22m ENOENT, no such file or directory 'C:\Users\rross\workspace\android-sencha-touch-2-phonegap-packaging\resources\images'
Everything seems to work fine, although i have a problem probably with the python script.
When i manually copy paste my sencha app on the android/assets/www folder the app runs fine.
But when i try to compile the project, only some of the sencha app files are copied into the "www" folder thus getting different kind of errors.
(like Uncaught TypeError: Object #<Object> has no method 'define)
←[1m←[31m[ERROR]←[39m←[22m ReferenceError: Can't find variable: deviceapis
Stack trace:
file:///C:/inetpub/wwwroot/myapp/cordova-2.0.0.js : 4132 : Anonymous
file:///C:/inetpub/wwwroot/myapp/cordova-2.0.0.js : 4114 : Anonymous
file:///C:/inetpub/wwwroot/myapp/cordova-2.0.0.js : 579 : Anonymous
file:///C:/inetpub/wwwroot/myapp/cordova-2.0.0.js : 619 : Anonymous
file:///C:/inetpub/wwwroot/myapp/cordova-2.0.0.js : 5648 : Anonymous
file:///C:/inetpub/wwwroot/myapp/cordova-2.0.0.js : 480 : Anonymous
file:///C:/inetpub/wwwroot/myapp/cordova-2.0.0.js : 579 : Anonymous
file:///C:/inetpub/wwwroot/myapp/cordova-2.0.0.js : 619 : Anonymous
file:///C:/inetpub/wwwroot/myapp/cordova-2.0.0.js : 78 : Anonymous
←[1m←[31m[ERROR]←[39m←[22m Failed loading your application from: 'file:///C:/ine
tpub/wwwroot/myapp/index.html'. Try setting the absolute URL to your application
for the 'url' item inside 'app.json'
I have finished the rest of the tutorial. My problem is that I cant see the changes I made in the Main.js . What could be the problem ? I save the file and refresh the project every time..
Good job on this tutorial, but I'm encountering a problem where I'm not able to find a solution for by myself.
I'm using Sensa Touch 2.1 and Sencha CMD v3.0.0.250.
I'm using your phyton sencha_wrapper to build the stuff, but my /assets/www is empty everytime.
Confuesing is, the script itself seems to run without encountering problems. (you can see the log here: http://pastebin.com/R37737LY).
Are there any common newb fails for this situation?
Thanks for the help :)
Regards :)
Thanks for the fast reply. Tested and it's not creating content within /assets/www as well. I also figured out, that the sdk folder is missing within my AppFolder. Maybe this is the problem? Or is this based in regards to using Sencha CMD and not the SDK Tool? :)
Thank's for the heads up!
If so, I guess I'll try to generate a new app and copy the content?
http://www.sencha.com/forum/showthread.php?248720-PhoneGap-Build-produces-broken-app-after-upgrading-from-ST-2.0.1-to-ST-2.1./page5
maybe you could help us
it worked on Android.
If I substitute the phonegap js to run on ios it doesn't work.
Any suggestion?
-"PhoneGap is set up almost as usual with Android projects, following the official guide, but some steps differ a bit. In the android folder:" When setting up phonegap. Do you create folders in phonegap directory or ST project directory?
-When you say "Make the changes to the main activity (in my case AndroidSenchaActivity)". What changes are you talking about and where is this AndroidSenchaActivity and how to I get to it?
-How do you actually know if PG is working? I get the sample project to come up in the website but I think that is just ST, not PG.
- I explicitly said it's the android folder. Have a look at the directory structure above, or on GitHub.
- Changes to the main activity: As documented in the PhoneGap guide I referenced.
- Check the section "Set up PhoneGap" again. In the last step you should test with the index.html that comes with the PhoneGap project template. If it runs correctly on the emulator, you can happily continue. You won't be able to test it in a normal browser until non-mobile OSes are supported – run it in the emulator to see whether PhoneGap's ondeviceready event fires, it will then show a blinking text or so.
"SENCHA BUILD FAILED, PLEASE CHECK FOR ERRORS AND RE-RUN BUILD (THIS LINE IS REMOVED AUTOMATICALLY IF SENCHA BUILD SUCCEEDS)
[ERROR] Failed to execute sencha command, did you reboot and ensure the sencha command is always on the PATH? ([Error 2] The system cannot find the file specified) "
I am getting this error can u suggest me where I am going wrong. every thing is configured correctly all are completely ok.
SENCHA BUILD FAILED, PLEASE CHECK FOR ERRORS AND RE-RUN BUILD (THIS LINE IS REMOVED AUTOMATICALLY IF SENCHA BUILD SUCCEEDS)
[ERROR] Failed to execute sencha command, did you reboot and ensure the sencha command is always on the PATH? ([WinError 2] The system cannot find the file specified)
Please help
I found personnly easier to have to 2 projects in Eclipse, one for Sencha code and another for Phonegap and have a simple builder (like a .bat file) in the Sencha one that call Sencha command (the output in complete in the Eclipse console view) and then copy the generated files to assets/www of the Phonegap project. I added in the builder settings the refresh of ressources, no need for touch.exe neither. Works great! Hope this help! Merry Christmas to all!
C:\Users\moss\Documents\NetBeansProjects\AndroidSencha>python sencha_wrapper.py app build testing -d android/assets/www
Running Sencha command...
[31m[1m[ERROR] The current working directory (C:\Users\moss\Documents\NetBeansProjects\AndroidSencha) is not a recognized Sencha SDK or application folder[22m[39m
Command failed
[31m[1m[ERROR] The current working directory (C:\Users\moss\Documents\NetBeansProjects\AndroidSencha) is not a recognized Sencha SDK or application folder[22m[39m
I followed your tutorial. i am using windows pc-32bit and python is not installed on my pc.so 'python' command not working on command prompt.I also tried "sencha app build testing -d android/assets/www ".it is also showing error(building fail)
I cant move ahead from here..
"python sencha_wrapper.py app build testing -d android/assets/www"
i get an Error
i checked my /assets/www folder
it had all the files except the index.html file
please help !!
where i'm going wrong.
i have followed the tutorial well
@Andreas: Thanks for the detailed tutorial. You should point out that people need a SASS compiler installed to help with some of the issues encountered while running the sencha_wrapper.py script.
SENCHA BUILD FAILED, PLEASE CHECK FOR ERRORS AND RE-RUN BUILD (THIS LINE IS REMOVED AUTOMATICALLY IF SENCHA BUILD SUCCEEDS)
[ERROR] Failed to execute sencha command, did you reboot and ensure the sencha command is always on the PATH? ([WinError 2] The system cannot find the file specified)
[ERROR] Failed to execute sencha command, did you reboot and ensure the sencha command is always on the PATH? ([WinError 2] The system cannot find the file specified)
i am geting this error in AndriodManifest.xml ,please give me a solution
1- i'm a bit confuse about project structure
2- when i download the application at Github , then extract it , i get error
please advice , thanks a lot
but when i run" python sencha_wrapper.py app build testing -d android/assets/www" command , at the command line i see "running sencha command ..."
and at eclipse :
SENCHA BUILD FAILED, PLEASE CHECK FOR ERRORS AND RE-RUN BUILD (THIS LINE IS REMOVED AUTOMATICALLY IF SENCHA BUILD SUCCEEDS)
[ERROR] Failed to execute sencha command, did you reboot and ensure the sencha command is always on the PATH? ([Error 2] The system cannot find the file specified)
how to fix this issue please
thanks again
Thank you for providing this info.
I checked out the code from git hub and try to import it on eclips , it open perfectly and i got the same application once I run it, but i could not able to do the edit part since those files are not available on eclips project. Could you please help me on this.
The ones on the Cordova site do not mention the specifics.
I have call it and it takes on and on (I saw that you wrote it colud take a while, but 20+mins)..nothing happens, when I try to open it via http://192.168.1.2:8000 I only get the files in the directory like there in no index.html file. Can u help me please, what am I missing? Thanks!